Java programming: Java program code consists of instructions which will be executed on your computer system to perform a task as an example say arrange given integers in ascending order. This page contains sample programs for beginners to understand how to use Java programming to write simple Java programs. These programs demonstrate how to get input from a user, working with loops, strings, and arrays. Programs are provided with the output (image file), and you can also download class file and execute it directly without compiling the source file.
public static void main(String[] args) {
int sum=0;
int i;
for(i=1;i<=10;++i)
{
System.out.println(" "+i);
sum=sum+i;
}
System.out.println("sum is"+" "+sum);
}
}
OUTPUT:
FOR BEGINERS.
Java programming examples
Example 1: Display message on computer screen.
- class First {
- public static void main(String[] arguments) {
- System.out.println("Let's do something using Java technology.");
- }
- }
This is similar to a hello world Java program.
Download java programming class file.
Download java programming class file.
Output of program:
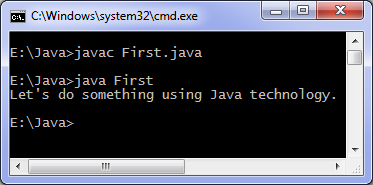
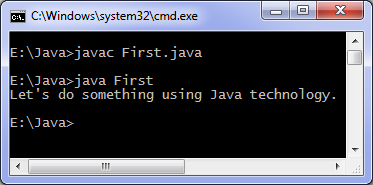
Example 2: Print integers
- class Integers {
- public static void main(String[] arguments) {
- int c; //declaring a variable
- /* Using for loop to repeat instruction execution */
- for (c = 1; c <= 10; c++) {
- System.out.println(c);
- }
- }
- }
Output:
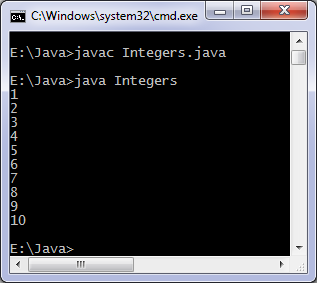
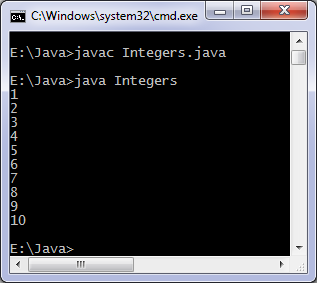
If else control instructions:
- class Condition {
- public static void main(String[] args) {
- boolean learning = true;
- if (learning) {
- System.out.println("Java programmer");
- }
- else {
- System.out.println("What are you doing here?");
- }
- }
- }
Output:
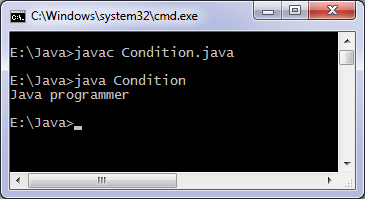
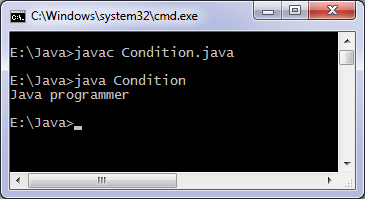
Command line arguments:
- class Arguments {
- public static void main(String[] args) {
- for (String t: args) {
- System.out.println(t);
- }
- }
- }
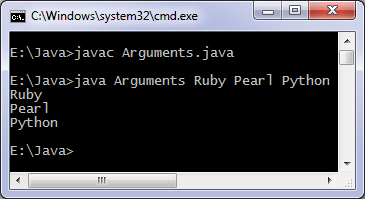
Java Development IDE
As your programming experience grows in Java you may be developing your own project or software, using a simple text editor isn't recommended. Following are two popular and open source IDE's:
Using IDE helps you a lot while coding as they offer many useful features such as you can create GUI in Netbeans without writing any code, Netbeans will show you any compilation error before you compile your code and it can also show hints on how to fix that.
Java programming tutorial
Java technology has changed our life as most of the devices we use today includes java that's why to learn java programming is a good thing. Java was developed by Sun Microsystems but now owned by Oracle. Here is a quick java tutorial for beginners, Java is an object-oriented computer programming like C++, if you already know C++ or any other object-oriented language, then it will be easier for you to learn java. Java program consists of classes which contain methods; you can't write a method outside of a class. Objects are instances of classes. Consider the following code:
- class ProgrammingLanguage {
- //attributes
- String language_name;
- String language_type;
- //constructor
- ProgrammingLanguage(String n, String t) {
- language_name = n;
- language_type = t;
- }
- //main method
- public static void main(String[] args) {
- //creating objects of class
- ProgrammingLanguage C = new ProgrammingLanguage("C", "Procedural");
- ProgrammingLanguage Cpp = new ProgrammingLanguage("C++", "Object oriented");
- //calling method
- C.display();
- Cpp.display();
- }
- //method (function in C++ programming)
- void display() {
- System.out.println("Language name:"+language_name);
- System.out.println("Language type:"+language_type);
- }
- }
There is a Programming Language class, and all programming languages will be instances of this class. We have considered only two attributes language name and type; we can create instances of the class using "new" keyword. There is a constructor method which is invoked when an object of a class is created we use it to name the programming language and its type. "Main" method is a must and acts as a starting point of a program, "display" method is used to print information about programming language object. Class names in Java begin with a capital letter, and if there are more words in class then their first letter will also be capital. For example, MyJavaClass is a class name, and for methods (functions in C or C++) the first letter is small and other words first letter is capital as an example myJava is method name. These are only conventions but are useful in distinguishing classes from methods. Java has a very rich API to build desktop and web applications.
No comments:
Post a Comment